Variables#
Introduction#
Python stores data in variables. To assign data to a variable we use the = sign.
For example,
x = 'Alison'
One way to think of variables is to treat them like a box. In the above example, we have a box named x, and the box is storing the value 10.
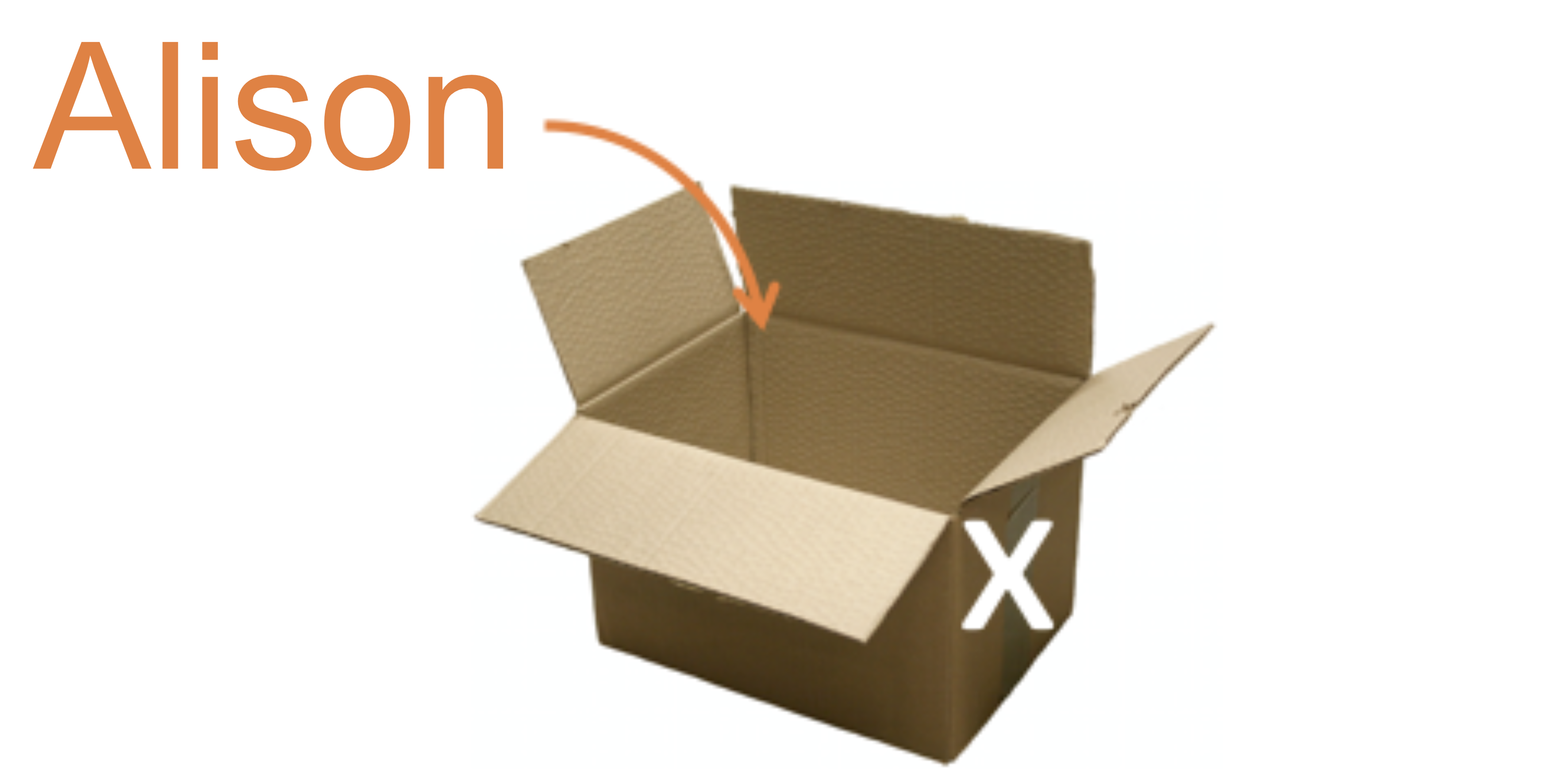
When we want to use the value x, we look for the box labelled x and extract the contents.
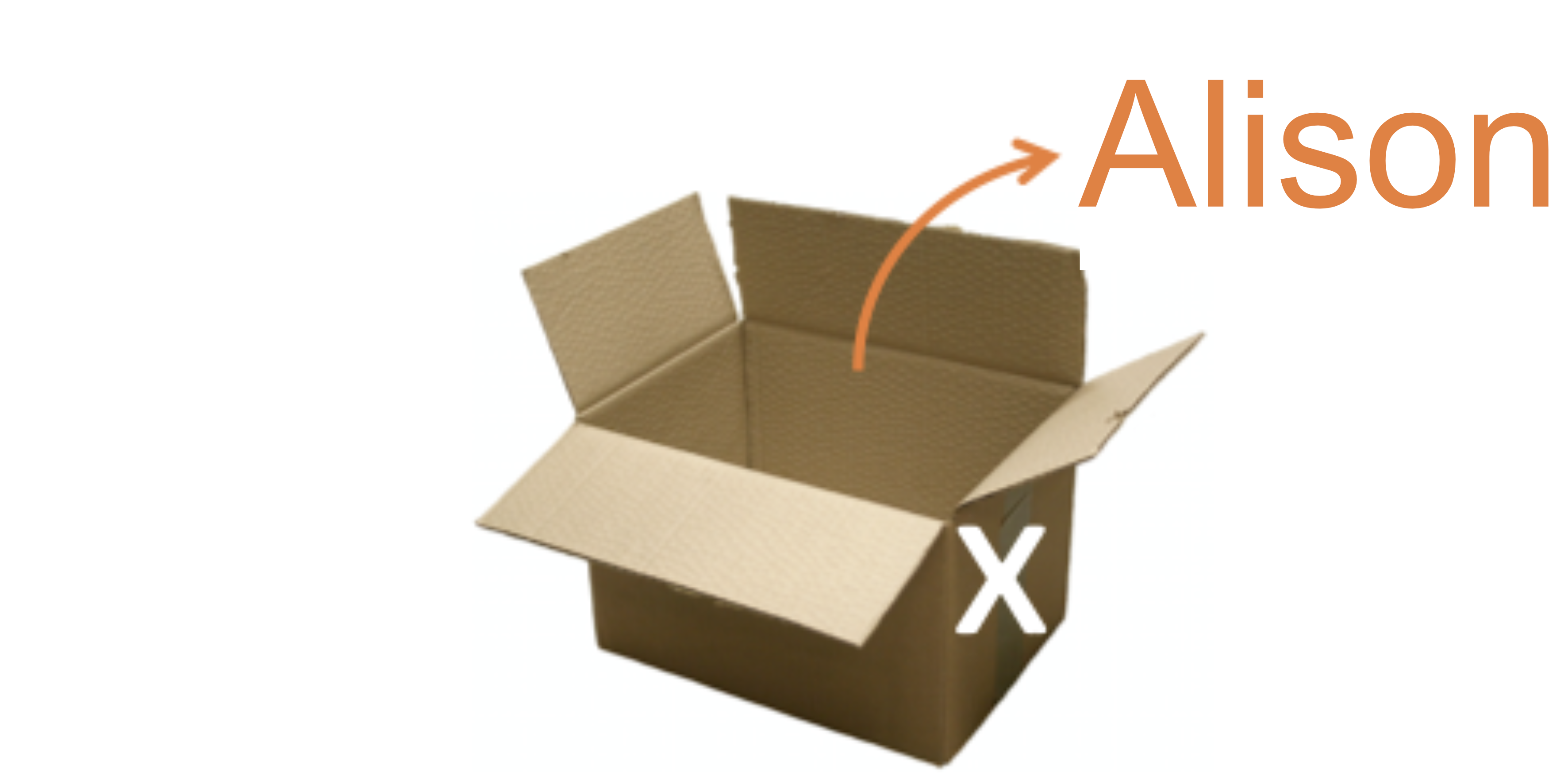
Here is an example:
x = 'Alison'
print(x)
Note that the variable x does not have quotes but the string ‘Alison’ does!
Types#
Python has different types of variables. Here are some of the most commonly used variables.
integer: a whole number
x = 5
num = 21
float: a decimal number
cost = 1.50
pi = 3.14159
string: text
name = 'steve'
message = "great job"
Note
You can use either single quotes ' '
or double quotes " "
to
indicate a string as long as you start and end with the same type.
char: a single character
letter = 'a'
symbol = '@'
Note
Python does not distinguish between characters and strings and will treat a single character as a string. But other languages will treat them differently!
If you want to know the type of a certain variable, we can use the
type
function.
print(type(3))
print(type(3.0))
print(type('3'))
<class 'int'>
<class 'float'>
<class 'str'>